What Does std Mean in C++? A Beginner-Friendly Guide to the Standard Namespace
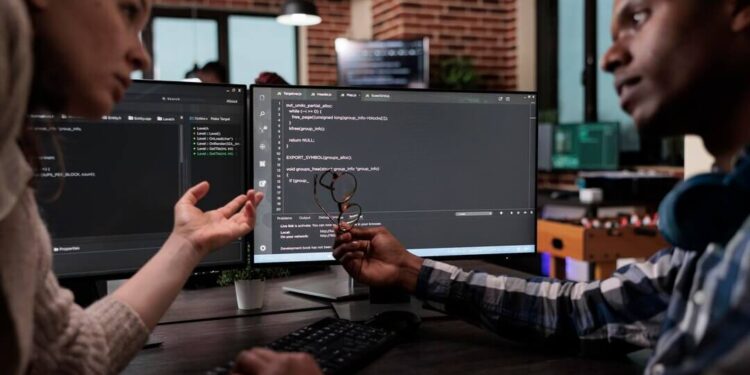
Introduction to C++ and the ‘std’ Keyword
When you start learning C++, one of the first things you’ll notice is the repeated use of the word std in code—like std::cout, std::cin, and std::string. At first glance, it might seem like cryptic jargon, but it’s actually a fundamental part of modern C++ programming.
So, what does std stand for in C++? Simply put, std is short for standard, and it refers to the Standard Namespace in C++. This namespace contains all the classes, functions, and objects provided by the C++ Standard Library.
Understanding std and namespaces in general is crucial because they prevent name conflicts, organize code, and help you use C++’s powerful built-in functionalities correctly and efficiently.
What is a Namespace in C++?
Before diving deep into std, it helps to understand what a namespace is. In C++, a namespace is a container that allows you to group identifiers—like variables, functions, and classes—under a name. This helps prevent naming collisions, especially in large programs or when using multiple libraries.
Here’s a quick example:
namespace MyNamespace {
int value = 10;
}
To access value, you’d use:
std::cout << MyNamespace::value;
So, when you see std::cout, you’re accessing the cout object inside the standard (std) namespace.
The Purpose of std in C++
The std namespace includes all the built-in functionality provided by the C++ Standard Library. This means:
- Input/Output operations: std::cout, std::cin, std::cerr
- Strings: std::string
- Data Structures: std::vector, std::map, std::set
- Utilities: std::swap, std::pair, std::make_pair
- Algorithms: std::sort, std::find, std::count
Without std, your code wouldn’t have direct access to any of these utilities. They’re all “organized” under this namespace to avoid clashing with custom variable names you might create.
Why Use std:: Instead of Just Writing cout or string?
Good question. You might wonder, “Why not just write cout instead of td::cout?”
The reason is name clarity and collision avoidance. Imagine you or someone else declares a function or variable named count or string—it could conflict with the version provided by the C++ Standard Library. Using std:: ensures you’re referencing the one from the standard library, not some custom-defined name.
However, for convenience, especially in small programs or teaching environments, you can use:
using namespace std;
This allows you to write:
cout << “Hello World!”;
instead of:
std::cout << “Hello World!”;
But be careful—using using namespace std; in large projects or headers is considered bad practice because it can pollute the global namespace and lead to ambiguity or errors.
Examples of Common std Components
Here are a few popular components from the std namespace that you’ll encounter often:
Component | What It Does | Example Use |
---|---|---|
std::cout | Prints output to the console | |
std::cin> | Reads input from the user | std::cin >> name; |
std::string | Represents text strings | std::string name = “Alice”; |
std::vector | A dynamic array that can grow/shrink in size | std::vector<int> nums; |
std::sort | Sorts elements in a container | std::sort(arr.begin(), arr.end()); |
How to Use std Properly in Your Programs
If you’re just starting out, you can simplify your code by writing:
using namespace std;
But as you move into more complex or collaborative coding, it’s better to explicitly use std:: for clarity and safety.
Here’s a full example using std correctly:
#include <iostream>
#include <string>
int main() {
std::string name;
std::cout << “Enter your name: “;
std::cin >> name;
std::cout << “Hello, ” << name << “!” << std::endl;
return 0;
}
Notice how each object from the Standard Library—cout, cin, string—is accessed using std::.
Conclusion: Why std is Essential in C++
To sum it up: std stands for standard, and it’s the namespace where all C++ Standard Library components live. Whether you’re working with input/output, strings, containers, or algorithms, you’ll be using std:: a lot.
While it might seem like an extra step to keep typing std::, it keeps your code organized, safe, and clear—especially as your programs get bigger and more complex.
So the next time you write std::cout << “Hello World!”;, you’ll know exactly what’s going on behind the scenes.